Friday, Nov 15, 2019
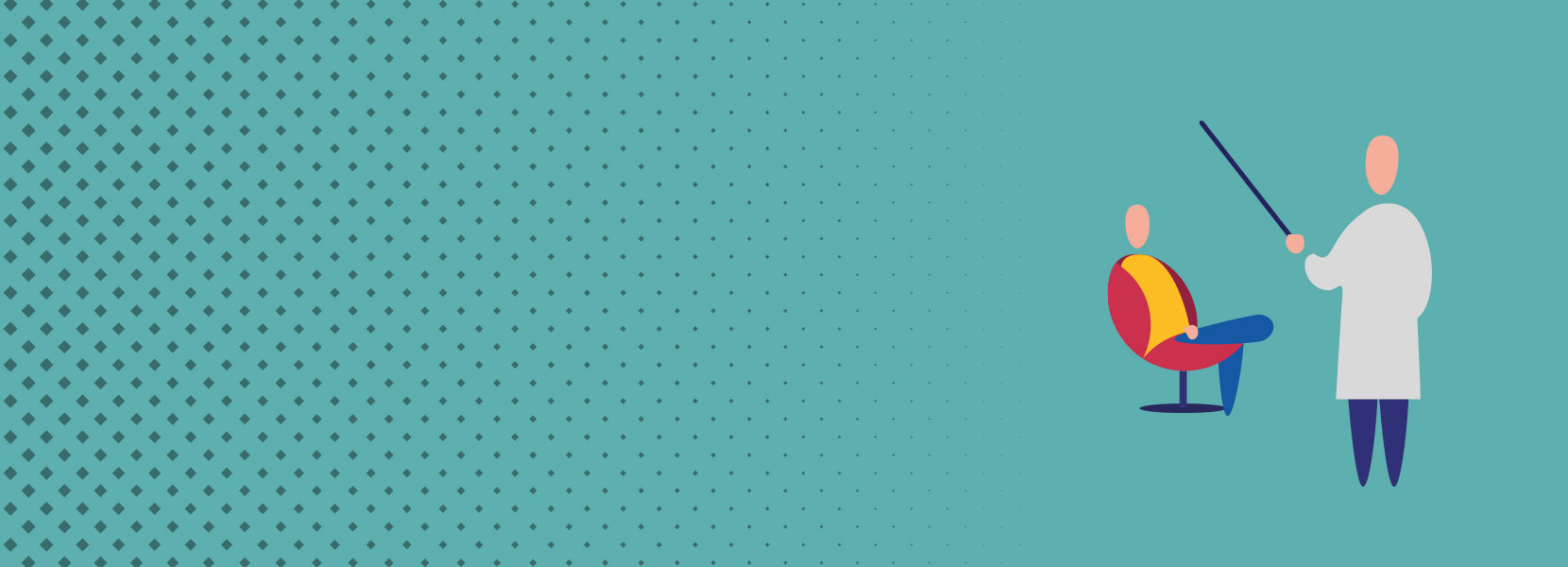
Advantages and disadvantages of micro-ORMs
What is an ORM?
For a start, let’s explain what an ORM is, what an ORM library is, and what sets micro and regular ORM libraries apart.
ORM stands for object-relational-mapping - a programming technique for converting data between incompatible systems. In practical terms, when referring to an ORM, we usually refer to an ORM library which does all the mapping from the objects defined in the code to a relational data model we would find in a relational database.
Some of the most commonly used ORM libraries in .NET are NHibernate, LLBLGen, and Microsoft’s Entity Framework.
ORMs allow you to query the database from the code so instead of writing SQL(Structured Query Language) directly inside your program, you would be able to write something like this for inserting an entity into an SQL database:
public DbSet<CategoryEntity> categories;
public void Insert(CategoryEntity categoryEntity)
{
categories.Add(categoryEntity);
}
This helps writing clean and straightforward code and makes it possible to query the database without writing straight SQL (keep in mind the examples provided are purposefully simplified).
What is a micro-ORM?
So what exactly makes an ORM them micro? Well, ORMs are usually full-blown frameworks, handling SQL generation, validation, database connections, object mapping, etc. In short, they’re designed to handle all aspects of code to database interaction.
Micro-ORMs, on the other hand, are much more hands-off in their approach. They focus only on mapping the rows from the database into your code representation of the data. The rest is left for the developer to handle.
Here is an example of inserting a simple Category
entity into an SQL database using the Dapper framework.
public Task Insert(Category category)
{
using (IDbConnection db = _dbConnection.SqlConnection)
{
var query = @"INSERT INTO category(id, name) VALUES(@id, @name)";
return db.ExecuteAsync(query, category);
}
}
As you can see, we’re querying the database using a parameterized query in C# code.
So, if micro-ORMs throw out a large amount of functionality that regular ORMs provide, why should we use them? Let’s go over some of their advantages to get a better picture.
The advantages
-
Micro-ORMs will usually perform better. Focusing on just mapping the database data to its code representation means less time is being spent doing other things - thus improving the performance. As well as being faster due to their minimalism, micro-ORMs also give control over hand-writing your queries. That opens up many possibilities in writing optimized SQL compared to relying on the auto-generated SQL by regular ORMs.
-
Setting up a full-blown ORM framework in your project might be a bit too much if your project only has a couple of database tables with data. For smaller projects, like small utilities, writing SQL queries in code probably won’t be a burden and will be far quicker to get up and run.
-
If your application makes heavy use of stored procedures or views, you might find that micro-ORMs leave your code simpler and easier to maintain. Since the logic is contained in the database, you don’t really need all the functionality that comes with full-blown ORM frameworks.
Now let’s go over some of the negatives.
The disadvantages
-
Having to write SQL queries in code directly might work when building a simple prototype/application or in isolated places where optimized SQL queries will be used for performance; but for a typical application, directly written queries can be cumbersome to maintain. In a large enterprise application, for example, you might find that using a micro-ORM would significantly hinder your ability to refactor your code.
-
Working with relationships can be a pain. Micro-ORMs work well with single objects, but for loading relationships on your objects, you’ll have to structure your queries in a special way.
-
If your application requires extensive validation, you’re better off sticking with regular ORMs. Micro-ORMs focus on mapping and performance at the cost of features like validation.
Should I switch to micro-ORMs?
As with everything, it depends. Be sure to carefully assess your requirements before making a choice which could potentially cost you lots of time and money down the road.
If you’re making a small utility application or your application is performance critical in places, then using a micro-ORM could be a good move. Other than that, it might be safer to stick to the regular ORM frameworks.